A buffer resource is a collection of fully typed data grouped into elements. You can use buffers to store a variety of data, including position vectors, normal vectors, texture coordinates in a vertex buffer, indexes in an index buffer, or device state. A buffer element consists of 1 to 4 components. Buffer elements can contain packed data values, single 8-bit integers, or four 32-bit floating point values. Buffer often plays an important role in 3D configurator projects.
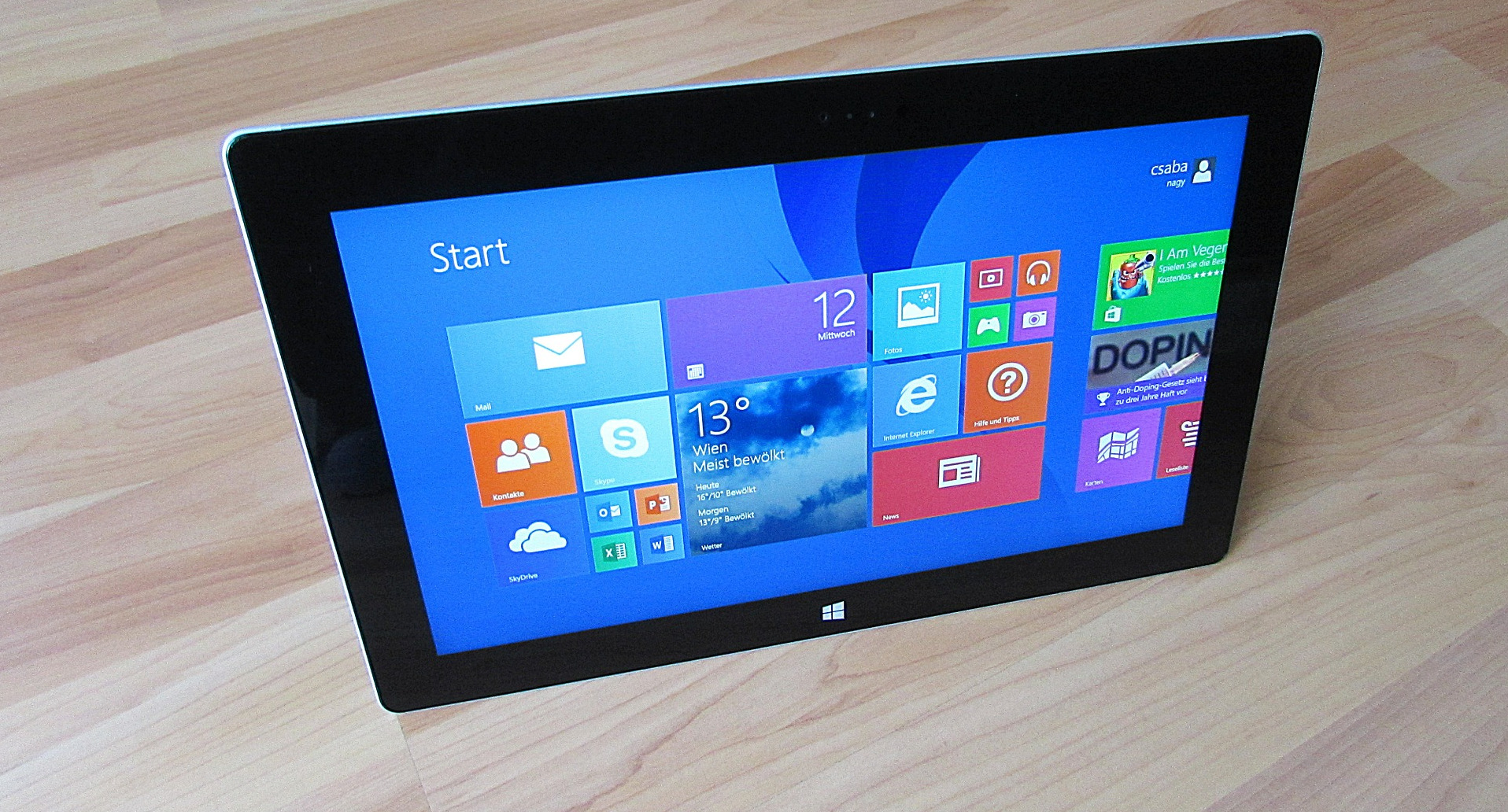
A buffer is created as an unstructured resource. Since it is unstructured, a buffer cannot contain any Mipmap layers, cannot be filtered during reading, and cannot be sampled multiple times.
Buffer types.
The buffer resource types supported by Direct3D are listed below. All buffer types are encapsulated by the ID3D11 buffer interface.
Vertex buffer.
A vertex buffer contains the vertex data used to define your geometry. Vertex data includes position coordinates, color data, texture coordinates, normal data and so on.
The simplest example of a vertex buffer is one that only contains position data.
More often, a vertex buffer contains all the data needed to fully specify 3D vertices. An example of this could be a node buffer containing pro-vertex positions, normal and texture coordinates. This data is usually organized as sets of per-vertex elements.
This vertex buffer contains per-vertex data. Each vertex stores three elements (position, normal and texture coordinates). The position and the normal value are typically indicated by three 32-bit floats (DXGI_FORMAT_R32G32B32_FLOAT) and the texture coordinates by two 32-bit floats (DXGI_FORMAT_R32G32_FLOAT).
To access data from a vertex buffer, you must know which vertex to access and the following additional buffer parameters:
Offset – the number of bytes from the beginning of the buffer to the data for the first node. You can specify the offset using method ID3D11DeviceContext:IASetVertexBuffers.
BaseVertexLocation – the number of bytes from the offset to the first node used by the corresponding Draw call.
Before you create a vertex buffer, you must define its layout by creating an ID3D11InputLayout interface. This is done by calling the method ID3D11Device::CreateInputLayout. After the Input Layout object is created, you can bind it to the Input Assembler phase by calling the ID3D11DeviceContext::IASetInputLayout method.
To create a vertex buffer, call ID3D11Device::CreateBuffer.
Index Buffer.
Index buffers contain integer offsets in vertex buffers and are used to represent primitives more efficiently. An index buffer contains a sequential set of 16-bit or 32-bit indexes. Each index is used to identify a node in a node buffer.
The sequential indexes stored in an index buffer have the following parameters:
Offset – the number of bytes from the base address of the index buffer. The offset is passed to the ID3D11DeviceContext::IASetIndexBuffer method.
StartIndexLocation – specifies the first index buffer element from the base address and the offset specified in the IASetIndexBuffer. The starting point is passed to the method ID3D11DeviceContext::DrawIndexed or ID3D11DeviceContext::DrawIndexedInstanced and represents the first index to be rendered.
IndexCount – the number of indexes to render. The number is passed to the DrawIndexed method.
Start of the Index Buffer = Index Buffer Base address + Offset (Bytes) + StartIndexLocation * ElementSize (Bytes).
In this calculation, ElementSize is the size of each index buffer element, which is either two or four bytes.
To create an index buffer, call ID3D11Device::CreateBuffer.
Constant Buffer.
A constant buffer allows you to efficiently provide shader constant data to the pipeline. You can use a constant buffer to store the results of the stream output stage.
Each element stores a 1:4 component constant determined by the format of the stored data. To create a shader constant buffer, call ID3D11Device::CreateBuffer and specify the member D3D11_BIND_CONSTANT_BUFFER of enumerate type D3D11_BIND_FLAG.
A constant buffer can only use a single Bind flag (D3D11_BIND_CONSTANT_BUFFER) that cannot be combined with another Bind flag. To bind a shader constant buffer to the pipeline, call one of the following methods: ID3D11DeviceContext::GSSetConstantBuffers, ID3D11DeviceContext::PSSetConstantBuffers, or ID3D11DeviceContext::VSSetConstantBuffers.
To read a shader constant buffer from a shader, use an HLSL load function (e.g. Load). Each shader level allows up to 15 shader constant buffers. Each buffer can hold up to 4,096 constants.
Today we are so far through with our article about buffer types in Direct3D. If you have any questions or suggestions, please feel free to contact our experts in our forum.
Thank you very much for your visit.
Leave A Comment