The advantages and disadvantages of different animation technologies.
Which animation tool is recommended? We hear this question very often in practice. Animation tools play a very important role in the creating process of a 3D configurator.
After working with a number of them, we can only say that there is no correct answer. It’s a complicated question and the answer is correspondingly complicated. In the following article we would like to clarify what you should use and when so that you can work with the right tool in every situation.
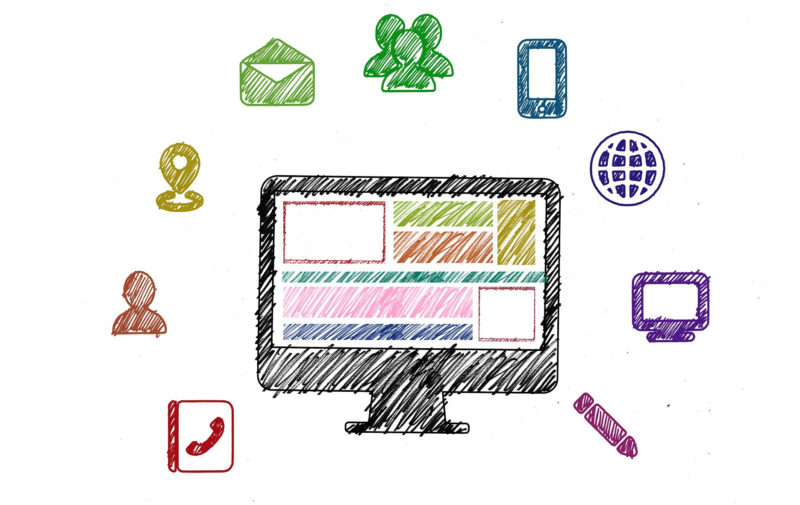
Of course we don’t have the possibility to go through every single animation library, so here we will focus on those that we have already used in practice and that are particularly interesting. Please note that these are our recommendations, which are based on our experience and may therefore differ from your experience.
Native Animation.
Before we talk about any libraries, let’s go through some native animation implementations.
CSS.
CSS remains one of our preferred ways to animate. We have been using it for years and are still excited about it because of its excellent performance. CSS animations allow the transition between different states using a number of keyframes.
Advantages:
- You do not need an external library.
- Performance is great, especially if you’re doing things that are hardware-accelerated by nature.
- Preprocessors (like Sass or Less) allow you to create variables (e.g. for easying functions or timings) that you want to keep consistent, along with :nth-child
- pseudo classes in functions to create amazing effects.
- You can listen to onAnimationEnd and some other animation shooks with native JavaScript.
- Movement along a path comes through the pipeline.
- It’s easy to use to speed up development as you can modify your animation with media queries.
Disadvantages:
- The Bezier Easings can be somewhat restrictive. With only two handles to shape the Bezier, you can’t create complex physical effects that are beautiful for realistic movements (but not very often necessary).
- If you go beyond chaining three animations in a row, it is recommended to switch to JavaScript. Sequencing in CSS becomes complex with delays, and you’ll end up with a lot of recalculations if you miss the timing. Staggers are also easier to write in JavaScript. You can latch into the native JavaScript events I mentioned earlier to work around this, but then switch the context between languages, which isn’t ideal either. Long, complex, sequential animations are easier to write and read in JavaScript.
- The support for movements along a path is not yet fully developed. We have registered to receive a bug report and requested a motion path module in CSS as a feature.
- CSS + SVG animation has a strange behavior. An example of this is that Safari browsers can combine opacity and transformations or have strange effects. Another reason is that the definition of the transformation origin by the specification, when applied sequentially, can appear in a non-intuitive way. It is the way the specification is written. Hopefully SVG 2 will help, but right now CSS and SVG on mobile devices sometimes need strange hacks to work properly.
requestAnimationFrame.
requestAnimationFrame (rAF) is a native method that is available on the Window object in JavaScript. It is very useful because it finds out what the right frame rate is for your animation in any environment you are in, and only drives it to that level. For example, if you are on a mobile device, the frame rate will not be as high as on the desktop. It also stops when a tab is inactive to prevent unnecessary use of resources. For this reason, requestAnimationFrame is a really high-performance way of animating and most of the libraries we will be dealing with use it internally. RequestAnimationFrame works in a similar way to recursion, before drawing the next frame in a sequence, it executes the logic and then calls itself again to continue. This may sound a little complex, but what it really means is that you have a set of commands that are executed all the time, so it will interpolate how the intermediate steps are represented very nicely for you.
Canvas is excellent for setting pixels in motion. So many of the impressive animations you see on CodePen are developed by canvas experts. As the name suggests, canvas provides visual space scripting where you can create complex drawings and high performance interactions. We work with pixels here, which means they are rasterized (as opposed to vectors).
Advantages:
- Since we don’t move things in the DOM, but only a pixel block, you can realize a ton of complex things without compromising performance.
- Canvas is really beautiful with interaction. You’re already in JavaScript, which gives you a lot of control without changing context.
- The performance is very good. Especially considering what you can do.
- The sky is the limit of what you want to create.
Disadvantages:
- It is difficult to make accessible. You’d have to use something like React to create a DOM for you, which Flipboard did recently, although in their case study they seemed to have more work to do. There’s probably an opportunity there if you’re interested in taking responsibility.
- We find it rather unintuitive to respond. By that we don’t mean that a small version works on the phone, because that’s rather positive. We mean moving and reorganizing content based on the viewport. We’re sure there are some really great developers out there who make it easy, but we’d say it’s not very easy.
- It’s not ready for retina. Unlike the SVG animation, which is resolution-independent, most canvas animations we’ve seen aren’t sharp on retina displays.
- It can be difficult to debug. If what you build no longer works, it breaks by showing nothing, unlike animation in the DOM, where things are still there, but just behave strangely.
SMIL.
SMIL is the native SVG animation specification. It allows them to move, morph, and even interact with SVGs directly in the SVG DOM. There are a lot of advantages and disadvantages to working with SMIL, but some factors will make us omit it altogether. It is increasingly losing acceptance.
Web Animations API.
The Web Animations API is native JavaScript that allows you to create complex sequential animations without loading external scripts. Or it will, as support grows. For now, you’ll probably need a polyfill. This native API was created as a backfill from all the great libraries and works that people have already done with JavaScript on the web. This is part of a movement that combines the power of CSS animation and the flexibility of sequencing in JavaScript under one roof.
Advantages:
- Sequencing is simple and easy to read.
- The performance seems to be on the right track. We haven’t tested it ourselves yet, but we are planning it (you can and should do your own performance tests).
Disadvantages:
- Support isn’t great right now, it’s still changing a bit, so you should be careful to run it in a production environment.
- There are still many things on the timeline in GSAP (which we’ll treat soon) that are more powerful. The important ones for us are the cross-browser stability for SVG and the ability to animate large sequences in a line of code.
WebGL.
There are some extraordinary things you can do with WebGL. If you’ve got something that’s socking you up, there’s a good chance it was created with WebGL. What it really does well are interactive 3D effects. Take a look at some demos. Technically it uses canvas.
Advantages:
- Impressive visual effects.
- Three dimensions mean a whole new world of interaction.
- Hardware-accelerated.
- Possibilities for VR.
Disadvantages:
- More difficult to learn than the other native animations we’ve covered so far. With the first two animations we made, we thought they wouldn’t work because the camera was pointing in the wrong direction. There are also very few good books and articles that explain how to work with them.
- Hard to answer. Most of the websites we’ve seen using WebGL have a “Please view this website on a desktop” hint.
External Libraries.
GreenSock (GSAP).
GreenSock is by far our favorite library we have worked with. Especially for SVG GSAP is recommended. The GreenSock Animation API has almost too many advantages to list you completely here. There are also good learning opportunities through forums and documents.
Advantages:
- It is extremely performant for something that is not native.
- The sequencing tools (like Timeline) are as easy to read as they are to write. It’s very easy to customize the timing, start multiple things simultaneously with relative names, and even start or stop your animations with a single line of code.
- You have a lot of other plugins if you want to create fancy things like animated text, morph SVGs or character paths.
- It solves SVG cross-browser problems especially for mobile devices.
- They offer really nice, very realistic simplifications.
- Since you can put any two integers in between, you can do cool things like animate SVG filters for some great effects and even work on canvas. There are almost no limits to what you can animate.
- GSAP is the only animation library, including the native SMIL, that lets you morph path data with an uneven number of points. This has opened up new possibilities for movement.
Disadvantages:
- You must pay for licensing if you resell your product to multiple users.
- As with any external library, you must look at which versions you are using in production. When new versions are released, the upgrade will require some testing (as with any library). You will also need to consider the size of the library.
VelocityJS.
VelocityJS provides much of the sequencing that GreenSock does. We no longer use Velocity due to a number of disadvantages. The syntax of Velocity looks a bit like jQuery, so if you’ve already used jQuery, you’ll be familiar with it.
Advantages:
- Linking many animations is much easier than with CSS.
- For refinements in eases there are step-eases in which you can pass an array.
- The documentation is pretty nice, so they are easy to learn and set up.
Disadvantages:
- The performance is not good. Despite some assertions to the contrary, we find that it doesn’t really hold when we run our own tests. We suggest that you run your own as the Internet is constantly changing.
- GSAP has more to offer for performance and cross-browser stability with no added weight. jQuery has lost performance testing in the past, but that may have changed since the introduction of the RAF, so Velocity is not bad at all, but it’s doing rather well in comparison.
- As far as it can be judged, it is no longer actively maintained. It is rumored that Julian Shapiro has passed the project on to someone else, so that it could revive sometime in the future.
jQuery.
jQuery is not only an animation library, but also has animation methods and is used by more and more users.
Advantages:
- Many websites have already loaded it, so it is usually easily accessible.
- The syntax is really easy to read and write.
- You have recently switched to requestAnimationFrame, so in versions 3.0.0 and above the performance will be better than before.
Disadvantages:
- Versions prior to 3.0.0 are particularly powerful and are not recommended. We have tested this very formally, but apart from the fact that you can see repaints and painting in Chrome DevTools, you can also see it with your eyes.
- Animations are not supported in SVG in any version.
- jQuery is limited to the DOM, unlike tools like GSAP which work in everything.
Snap.svg
Many people think Snap is an animation library, but it was never intended to be one. We wanted to do performance benchmarks on Snap, but even Dmitri Baranovskij says here in the SVG Immersion Podcast that it’s not the right tool:
A little note from Baranovskij: “Snap is not an animation library and I always recommend to use it with GSAP if you need a serious animation”.
This means that jQuery doesn’t work well enough with SVG, although you now support class operations. If we have to do a DOM manipulation with SVG, Snap is really great for it.
A library called SnapFoo was recently released and it extends Snap’s realm for animation. I haven’t played it myself yet, but it looks pretty cool and is worth a try.
Mo.js
This is a library from a developer, which comes from LegoMushroom. It’s a highly talented animator and has already made some great demos for this library that really excited us. This library is still in beta and is very close to release.
Advantages:
- There are things like shapes, eruptions and swirls that are very easy to work with. With Mo.js you don’t have to be the best and most creative illustrator in the world to get something new going.
- Mo.js offers some of the best and most beautiful tools on the Internet, including players, timelines and custom path creators. That’s the main reason to use Mo.js.
- There are several ways to animate – one is an object, one draws an object. Change a lightness over time – so you can decide in which direction you feel more comfortable.
Disadvantages:
- It does not yet offer the possibility to use an SVG as a superordinate element for the user-defined shapes, so that working with coordinate systems and scaling for response is less intuitive and more difficult to do on mobile devices. However, this is a fairly advanced technique, so you may not need it.
- It doesn’t correct the cross-browser behavior we have GreenSock yet, which means you may need to write hacks, as is the case with CSS.
Three.js
Three.js is a wonderful three-dimensional animation tool. We regularly use Three.js for the development of our real-time 3D configurators and have only had positive experiences with it so far.
Bodymovin
Bodymovin is intended for creating animations in Adobe After Effects that you can easily export to SVG and JavaScript. Some of the demos are really impressive. We don’t work with them because we really like them. Creating things from scratch with code, but if you’re more of a designer than a developer, you’ll really like it. The only disadvantage is that if you have to change something later, you have to export it again. This could be a strange workflow. Also, the code output is usually kind of big, but we didn’t see that it affects performance too much in the case of Bodymovin, so it’s probably fine.
React-specific workflows.
Before we go into React, let’s talk about why we need to treat animations differently in React. DOM stands for Document Object Model. We create a structured document with objects whose nodes we call a tree. React interacts with a virtual DOM, which is an abstraction unlike the native browser implementation.
React uses a number of virtual DOM reasons, the most compelling of which is the ability to find out what has changed and update only the parts it needs. This abstraction has its price and some of the old tricks you work with will give you trouble in a React environment. For example, iQuery will work fine with React because the primary function is to interact and manipulate with the browser’s native DOM. We have even seen some strange CSS race conditions.
React Motion.
React Motion is a very popular way to animate in React, and the community has adopted it through the old ReactCSSTransitionGroup, which used to be the most widely used.
React Motion is very similar to the playful animation where you give an element mass and physics and get it on its way and it arrives when it arrives. This means that you don’t specify a time span as you do with CSS or other traditional web-based sequential movements. This is a completely different way of thinking about animation. This can mean that the motion has the ability to look really realistic, which can be very nice. But user interfaces have different requirements than games, so working with them can be difficult. Let’s say you have two things that have to fire independently and get there at the same time that are different. It can be difficult to fine-tune them.
Until recently, there was no sequencing. Just last week Cheng Lou added Lou to onRest, which makes this kind of callback workflow possible. It is still not easy to write a loop without writing an infinite loop. You may not need this, but we thought it should at least not go unmentioned at this point. As FYI for the performance sensitive it uses rendering.
Advantages:
- The animation can look really nice and realistic and the spring options are impressive.
- The stunning effect is pretty good. It’s also available in most JS libraries, but it’s created based on the last move, not by duplicating the last, so there are some nice moves.
- The recording of onRest is a great thing. It allows some sequencing that wasn’t available before, so when you look at the demos you won’t even see everything they have to offer.
- I would currently consider this to be the animation tool that works best with React.
Disadvantages:
- It’s not a super plug-and-play like some other or native libraries, which means you end up writing a little more code. Some people like that about it, others don’t. It’s not good for beginners, though.
- Sequencing is not as easy and readable as some of the alternatives due to the complex nature of the code.
- OnRest does not yet work for staggering parameters.
GSAP in React.
GreenSock has so much to offer that it is still worth using it in a React environment. This is especially true for complex sequencing where React-Motion does not offer a lean solution and GreenSock shines. It takes a little more fine-tuning than usual and some things that should work and have to do with the DOM are not present in React.
- Get involved with the native methods of the React component life cycle.
- Create a function that has a timeline and then call that function within something you use for interaction. We can have an inline event handler we have onClick() and within the custom function, we will call another function that contains a timeline. In a jQuery or Vanilla JS setup, we would stop the timeline first and start playback, but we won’t do that here.
- You can use a library like React-GSAP-Enhancer. We haven’t worked with it too much ourselves, but there are really great demos.
CSS in React.
CSS-Animationen haben in letzter Zeit eine gewissen Wiederbelebung erfahren, da es der einfachste Weg ist, Animationen in React zu erstellen. Für kleine Animationen wir UI/UX-Interaktionen ist React hervorragend geeignet. Darüber hinaus eignen sie sich auch gut für kleine Anpassungen der Benutzeroberfläche.
Schlußfolgerungen.
Mit diesem Beitrag haben wir ihnen einen kurzen Überblick darüber gegeben, welche Technologien Sie für das Erstellen ihrer Animationen verwenden können. Es sind unsere persönlichen Erfahrungen, so dass andere Nutzer andere Erfahrungen gemacht haben können. Dennoch hoffen wir, ihnen einen ersten kurzen Überblick ermöglicht zu haben.
Vielen Dank für ihren Besuch.